How do software developers code efficiently
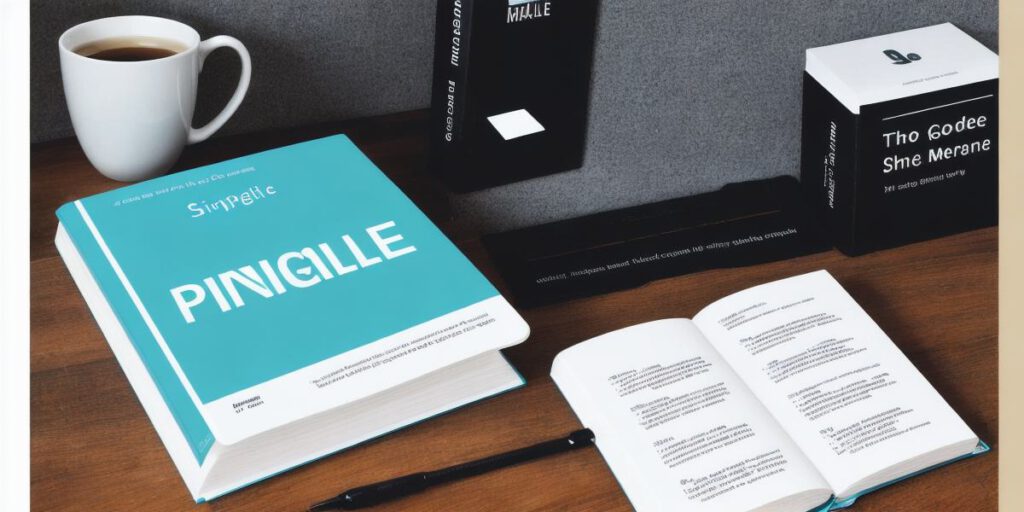
Introduction:
In the fast-paced world of software development, efficiency is crucial. Writing clean, concise, and well-structured code can save time, reduce errors, and improve overall performance. In this article, we will explore some of the best practices and techniques used by top developers to write efficient code. From code structure and organization to debugging and testing, these tips and tricks will help you streamline your development process and write better code.
Chapter 1: Code Structure and Organization
A well-structured and organized codebase is essential for efficiency and scalability. Here are some of the best practices for writing efficient code:
- Use meaningful variable names: Choose descriptive and concise names that accurately represent the purpose of each variable. This will make your code more readable and easier to understand, even for other developers. For example, instead of using a generic variable name like "x", use a more specific name like "userInput" or "total".
- Break up your code into small, modular functions: By breaking down your code into smaller, reusable functions, you can reduce redundancy, improve maintainability, and make it easier to debug your code. This approach also makes your code more readable and easier to understand, as each function has a specific purpose.
- Use comments sparingly: While comments are essential for documentation, they can also clutter your code and slow down the review process. Use comments only when necessary, and keep them concise and focused. For example, use comments to explain complex logic or to provide context for specific functions or variables.
- Follow the SOLID principles: The SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) are a set of guidelines for writing efficient and maintainable code. By following these principles, you can create cleaner, more scalable, and easier-to-maintain codebases. For example, the Single Responsibility principle encourages you to write functions that have only one reason to change, while the Open-Closed principle allows you to extend functionality without modifying existing code.
- Use design patterns: Design patterns are reusable solutions to common programming problems. By using design patterns, you can write more efficient and robust code that solves complex problems in a clear and concise way. For example, the Singleton pattern ensures that a class has only one instance and provides a global point of access to it, while the Factory pattern provides an interface for creating objects, but allows subclasses to determine which class to instantiate.
Chapter 2: Debugging and Testing
Debugging and testing are critical for identifying errors and ensuring the quality of your code. Here are some of the best practices for debugging and testing:
- Use automated testing: Automated testing can save time and reduce errors by catching bugs early in the development process. By using automated tests, you can write better, more reliable code that meets the requirements and performs well under different conditions. For example, you can use unit tests to test individual functions or methods, and integration tests to test how those functions work together.
- Debug with a clear understanding of the problem: Before diving into debugging, take some time to understand the problem and identify the root cause. This will help you focus on the most important aspects of the issue and avoid wasting time on irrelevant details. For example, if you are trying to debug a memory leak, start by identifying which part of your code is allocating memory, and then use tools like valgrind or gdb to identify the specific memory locations that are leaking.
- Use logging and tracing: Logging and tracing can provide valuable insights into the behavior of your code and help you identify issues that might be difficult to debug manually. By using logging and tracing, you can create a more robust and efficient debugging process. For example, you can use log4j or slf4j for Java applications, or Python’s built-in logging module for Python applications.
- Write unit tests: Unit tests are a type of automated test that focus on testing individual units of code, such as functions or methods. By writing unit tests, you can catch bugs early in the development process and ensure that your code is working as expected. For example, you can use JUnit for Java applications, or unittest2 for Python applications.
- Use continuous integration and deployment: Continuous integration and deployment (CI/CD) tools can automate the testing and deployment process, making it easier to identify and fix bugs, and ensuring that your code is always up-to-date and ready for production. For example, you can use Jenkins or Travis CI for Java applications, or CircleCI or GitLab CI/CD for Python applications.
Chapter 3: Optimization
Optimization is the process of improving the performance of your code to run faster and more efficiently. Here are some best practices for optimizing your code:
- Profile your code: Profiling is a technique for measuring the performance of your code and identifying bottlenecks. By profiling your code, you can optimize it for specific performance metrics and ensure that it meets your requirements. For example, you can use tools like JProfiler or VisualVM for Java applications, or cProfile for Python applications.
- Use data structures efficiently: The choice of data structure can have a significant impact on the performance of your code. Choose the data structure that best fits your needs, and use it efficiently. For example, if you need to perform frequent insertions and deletions from a list, use a doubly-linked list instead of an ArrayList.
- Optimize algorithms: The choice of algorithm can also have a significant impact on the performance of your code. Choose the algorithm that best fits your needs, and optimize it if necessary. For example, if you need to find the largest element in an array, use binary search instead of linear search.
- Avoid unnecessary computations: Unnecessary computations can slow down your code and reduce its efficiency. Avoid performing calculations that are not needed, and cache results when possible. For example, if you calculate a value multiple times, store it in a variable or a cache to avoid redundant calculations.
- Use multithreading and parallelism: Multithreading and parallelism can help improve the performance of your code by allowing it to run concurrently on multiple processors or cores. For example, you can use Python’s multiprocessing module for CPU-bound tasks, or OpenMP for C/C++ applications.
Summary:
Writing efficient code requires careful planning, attention to detail, and a commitment to best practices. By following the tips and tricks outlined in this article, you can streamline your development process, write better code, and build more efficient applications. Remember to stay curious, keep learning, and always strive for improvement. With these skills, you’ll be well on your way to becoming a top developer.